Sep 29, 2019 My first actual code: tic-tac-toe game for c. GitHub Gist: instantly share code, notes, and snippets. Skip to content. All gists Back to GitHub. Sign in Sign up Instantly share code, notes, and snippets. Boomshaka / tic-tac-toe. Last active Sep 29, 2019. Star 2 Fork 1. Jun 10, 2019 This tutorial explains how to create a simple Tic Tac Toe Game in the C programming language. Tic-tac-toe is a game where two players X and O fill the hash (#) shaped box with their alternate turns. The player who first fills the box with 3Xs or 3Os in a horizontal, vertical or diagonal manner will win the game. I'm coding a game of tic tac toe in C. Here's the logic. The User goes first, selects a slot. The positions of the slot are calculated using simple formulae. Then the selected slot is filled. Next, the computer selects a slot, randomly. For this, i've used the rand function, in stdlib.h. Initially, all elements of the 3x3 matrix are set at 0.
Project Creation
Html Tic Tac Toe Code
1: Project Setup On Windows2: Project Setup On Mac
Game Engine Setup
3: State Machine4: Asset Manager
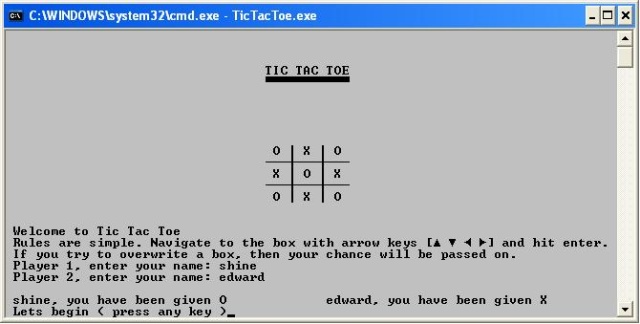
5: Input Manager
6: Game Loop
State/Screen Creation
7: Splash State/Screen8: Main Menu State/Screen
9: Game State/Screen
10: Pause State/Screen
11: Game Over State/Screen
Game Logic
12: Grid Part 1 - Initialisation13: Grid Part 2 - Placing A Piece
14: Grid Part 3 - Check For Win Condition
15: AI
16: Show Game Over Screen
Extras
17: What To Do Next?State Machine Based On: https://github.com/kiswa/SFML_Starter
Our assignment was to write a tic-tac-toe program for my programming class.
It is person vs. computer and the computer makes 'random' moves, even if computer is going to lose they will choose a random place to put their x or o
it is all correct, now what I have to do is modify the program so the computer plays 'smart', and by smart i mean blocking the player from making a 3 of a kind, etc.
so I have no idea how to do that
Tic Tac Toe Program Code
here is my program now:
- 4 Contributors
- forum 3 Replies
- 1,688 Views
- 4 Years Discussion Span
- commentLatest Postby Nayana sharmaLatest Post
VernonDozier2,218
Dev C++ Tic Tac Toe Source Code
I'd probably have an integer function called:
This function would test to see whether any move by the human opponent could win the game. It would return some number that is not 0 through 8 (i.e. -1) if there was no winning move that the player could make to win, and it would return some number from 0 to 8 if the player COULD make a winning move. The 0 through 8 return value would be the square representing the winning move. Call that function. If it returns -1, then call the makeRandMove function. If it returns something that is 0 through 8, don't call makeRandMove. Instead, have the computer's move be the return value from 'PlayerHasWinningMove'. That will block the player's move before he/she can make it.